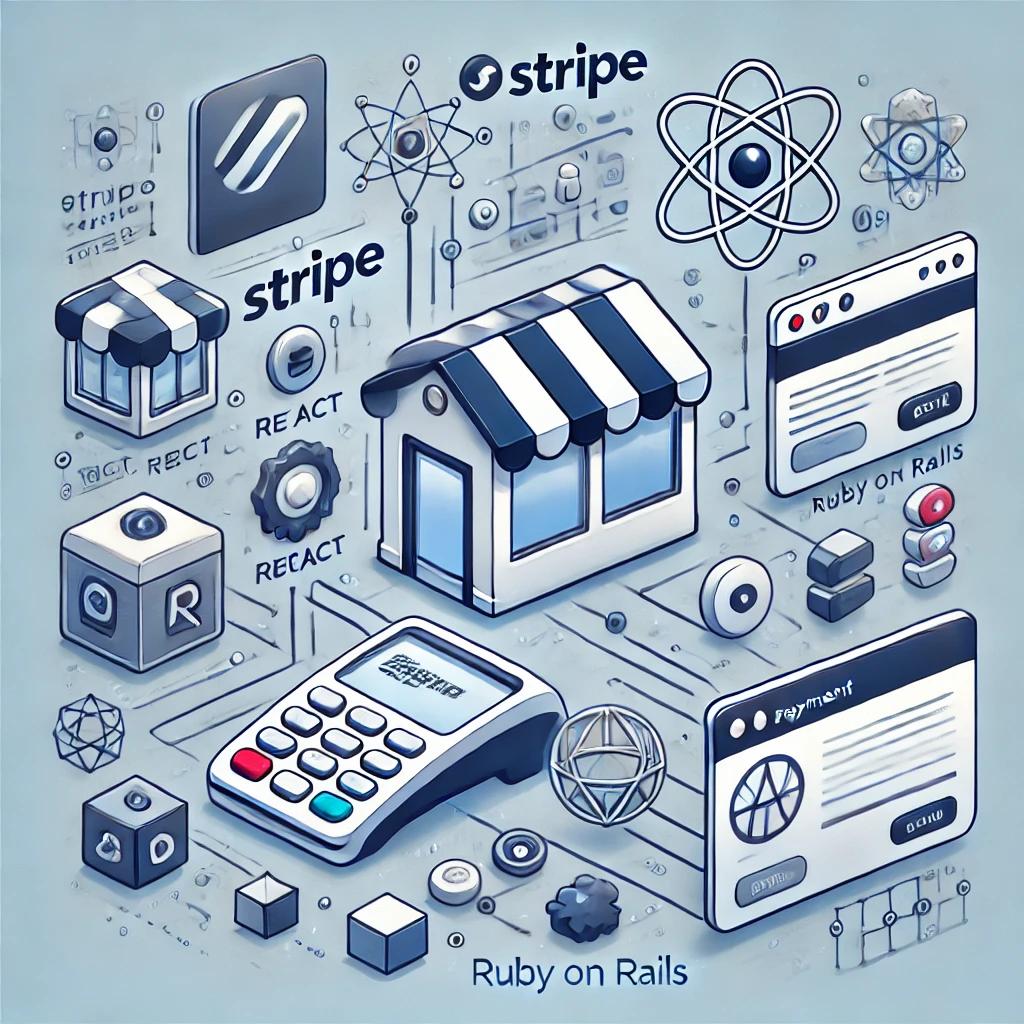
Introduction
In the world of digital transactions, Stripe stands out as one of the most versatile and widely used payment gateways. Known for its powerful API and easy integration, Stripe provides a seamless solution for businesses of all sizes, whether you’re running a small online store or a large-scale SaaS platform. Stripe’s global support, intuitive dashboard, and comprehensive documentation make it a go-to choice for developers and business owners alike.
What is Stripe and Why Use It?
Stripe is a payment processing platform that allows businesses to accept credit card payments and manage transactions online. Designed to simplify complex payment flows, it enables companies to process payments, manage subscriptions, and handle payouts—all while maintaining security and compliance standards. Stripe is particularly beneficial for:
- E-commerce platforms: Easy checkout integration for a smooth user experience.
- SaaS businesses: Simple management of subscriptions and recurring payments.
- Marketplaces: Facilitates multi-party payouts and transaction tracking.
Businesses favour Stripe for its ease of use, transparent pricing, and flexibility to work across a wide range of industries and platforms, making it an ideal solution for scaling online payment processes.
Countries Supported by Stripe
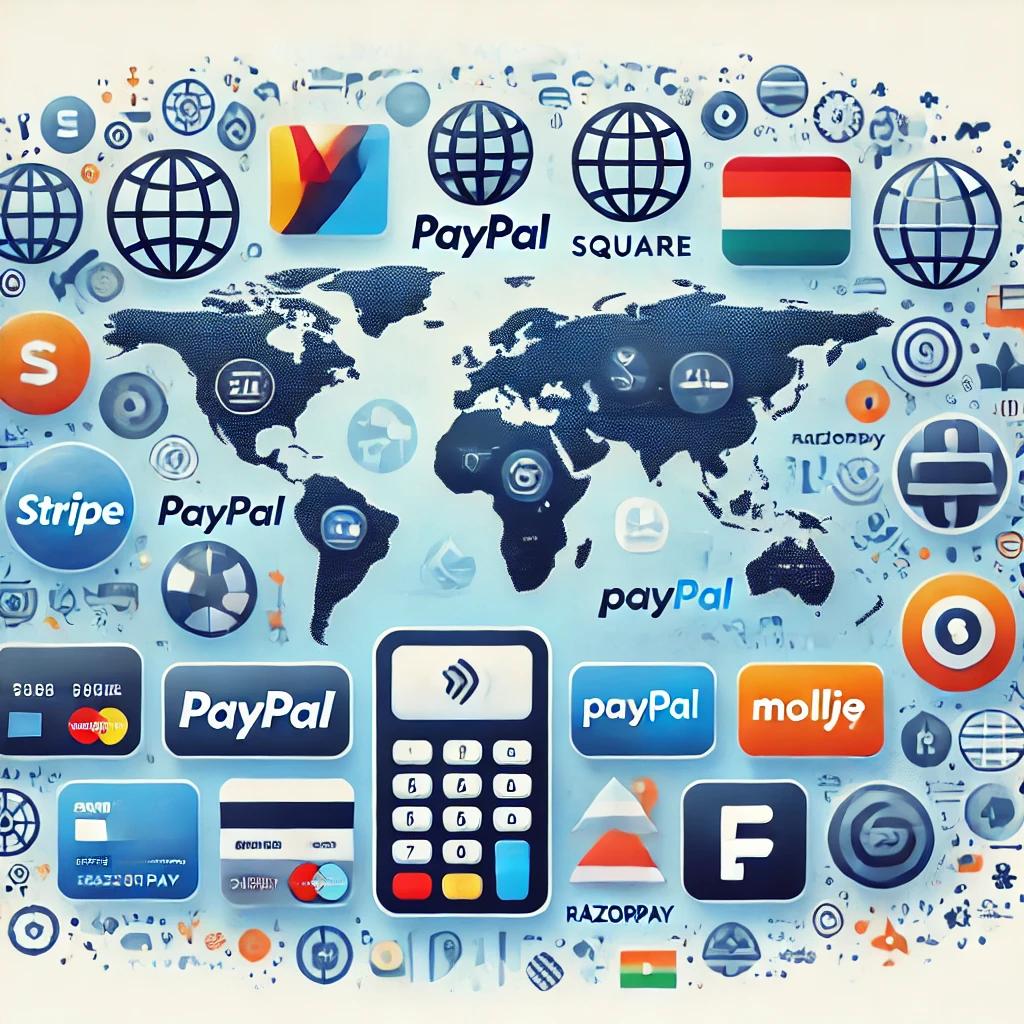
Stripe operates in more than 40 countries across North America, Europe, Asia-Pacific, and Latin America. Supported countries include the USA, Canada, most European Union countries, Australia, Japan, and Singapore, among others. However, some of Stripe’s advanced features may vary based on regional regulations, such as multi-currency support or local payment methods. For the latest list of supported countries, visit Stripe’s Global Availability page.
How to Integrate Stripe with Rails
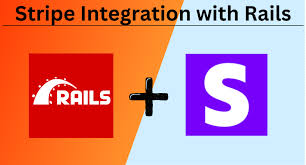
1. Setting Up Stripe in Rails
Integrating Stripe with Rails requires a few basic steps to get started:
1. Install the Stripe gem in your Rails application by adding it to your Gemfile
:
gem 'stripe'
2. Set up API keys: Sign up for a Stripe account and retrieve your API keys from the dashboard.
3. Configure your environment: Create a Stripe initializer in config/initializers/stripe.rb
to store your API keys.
Stripe.api_key = Rails.application.credentials.dig(:stripe, :secret_key)
# if you want to use credentials file for encryption and security
otherwise you can use environment variables also.
2. Implementing Stripe Payments
To create charges and manage subscriptions, you can use Stripe’s API to handle both single and recurring payments. Here’s a basic example for a one-time charge:
# Controller action
def create_charge
Stripe::Charge.create(
amount: 5000, # Amount in cents
currency: 'usd',
source: params[:stripeToken],
description: 'Sample Charge'
)
end
For subscriptions, you can use Stripe’s Subscription
object to create and manage recurring charges. Be sure to create a Product and Plan in your Stripe dashboard before implementing subscriptions.
Integrating Stripe with React
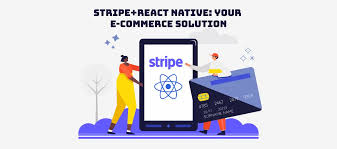
1. Using the Stripe API with React
To integrate Stripe with a React frontend, you’ll use the Stripe.js library, which allows for secure, PCI-compliant handling of payment information. Install the @stripe/stripe-js
package to start:
npm install @stripe/stripe-js
Set up Stripe with a secure checkout form:
import { loadStripe } from "@stripe/stripe-js";
const stripePromise = loadStripe("your-public-key");
async function handleCheckout() {
const stripe = await stripePromise;
const { error } = await stripe.redirectToCheckout({
lineItems: [{ price: "price_id", quantity: 1 }],
mode: "payment",
successUrl: window.location.origin + "/success",
cancelUrl: window.location.origin + "/cancel",
});
if (error) {
console.error(error);
}
}
2. Handling Subscription Models
With React, managing subscriptions involves creating a subscription form and using Stripe’s API to manage recurring payments. Here’s an example of setting up a subscription flow:
// Set up a subscription with Stripe
async function createSubscription() {
const response = await fetch("/create-subscription", {
method: "POST",
headers: {
"Content-Type": "application/json",
},
});
const session = await response.json();
const { error } = await stripe.redirectToCheckout({
sessionId: session.id,
});
if (error) {
console.error(error);
}
}
Comprehensive Overview of Stripe’s Features
Subscriptions & Recurring Payments
Stripe simplifies the setup for recurring payments and subscription management. To create subscriptions, use Stripe’s Products and Pricing Plans and apply them to customers using the Subscription API.
Charges and One-Time Payments
Processing one-time payments with Stripe is straightforward. Using the Charges API, you can handle direct payments with credit cards or other supported methods, which is ideal for e-commerce purchases.
Payouts and Withdrawal Management
Stripe’s payout process is automated, with earnings transferred to your bank account on a set schedule. Payout timelines vary by country and business type, and Stripe charges a small fee per payout. Managing your payout settings can help reduce fees and optimize cash flow.
Best Practices for Using Stripe Effectively
- Optimize Security: Always use Stripe’s PCI-compliant features like Stripe Elements or Stripe Checkout.
- Minimize Fees: Stripe charges a small transaction fee; consider batching payouts or using Stripe’s advanced features like Radar for fraud prevention.
- Automate Workflows: Stripe offers webhook support to automate refunds, failed payments, and other events.
Here’s a detailed blog post draft following your specifications:
Mastering Stripe for Web Payments: Setup, Features & Integrations
Meta Description
Explore Stripe’s features, country support, and setup guides for Rails & React. Learn about subscriptions, charges, payouts, alternatives, and top payment gateways.
Introduction
In the world of digital transactions, Stripe stands out as one of the most versatile and widely used payment gateways. Known for its powerful API and easy integration, Stripe provides a seamless solution for businesses of all sizes, whether you’re running a small online store or a large-scale SaaS platform. Stripe’s global support, intuitive dashboard, and comprehensive documentation make it a go-to choice for developers and business owners alike.
What is Stripe and Why Use It?
Stripe is a payment processing platform that allows businesses to accept credit card payments and manage transactions online. Designed to simplify complex payment flows, it enables companies to process payments, manage subscriptions, and handle payouts—all while maintaining security and compliance standards. Stripe is particularly beneficial for:
- E-commerce platforms: Easy checkout integration for a smooth user experience.
- SaaS businesses: Simple management of subscriptions and recurring payments.
- Marketplaces: Facilitates multi-party payouts and transaction tracking.
Businesses favor Stripe for its ease of use, transparent pricing, and flexibility to work across a wide range of industries and platforms, making it an ideal solution for scaling online payment processes.
Countries Supported by Stripe
Stripe operates in more than 40 countries across North America, Europe, Asia-Pacific, and Latin America. Supported countries include the USA, Canada, most European Union countries, Australia, Japan, and Singapore, among others. However, some of Stripe’s advanced features may vary based on regional regulations, such as multi-currency support or local payment methods. For the latest list of supported countries, visit Stripe’s Global Availability page.
How to Integrate Stripe with Rails
1. Setting Up Stripe in Rails
Integrating Stripe with Rails requires a few basic steps to get started:
- Install the Stripe gem in your Rails application by adding it to your
Gemfile
:rubyCopy codegem 'stripe'
- Set up API keys: Sign up for a Stripe account and retrieve your API keys from the dashboard.
- Configure your environment: Create a Stripe initializer in
config/initializers/stripe.rb
to store your API keys.rubyCopy codeStripe.api_key = Rails.application.credentials.dig(:stripe, :secret_key)
- Creating a Checkout Page
To build a checkout page, create a controller action that initializes a payment session.
def create_checkout_session
session = Stripe::Checkout::Session.create(
payment_method_types: ['card'],
line_items: [{
price_data: {
currency: 'usd',
product_data: {
name: 'Sample Product',
},
unit_amount: 5000,
},
quantity: 1,
}],
mode: 'payment',
success_url: success_url,
cancel_url: cancel_url
)
redirect_to session.url, allow_other_host: true
end
This code sets up a one-time payment session, redirecting customers to a secure Stripe-hosted checkout page.
2. Implementing Stripe Payments
To create charges and manage subscriptions, you can use Stripe’s API to handle both single and recurring payments. Here’s a basic example for a one-time charge:
rubyCopy code# Controller action
def create_charge
Stripe::Charge.create(
amount: 5000, # Amount in cents
currency: 'usd',
source: params[:stripeToken],
description: 'Sample Charge'
)
end
For subscriptions, you can use Stripe’s Subscription
object to create and manage recurring charges. Be sure to create a Product and Plan in your Stripe dashboard before implementing subscriptions.
Creating a Checkout Page
Integrating Stripe with React
1. Using the Stripe API with React
To integrate Stripe with a React frontend, you’ll use the Stripe.js library, which allows for secure, PCI-compliant handling of payment information. Install the @stripe/stripe-js
package to start:
bashCopy codenpm install @stripe/stripe-js
Set up Stripe with a secure checkout form:
javascriptCopy codeimport { loadStripe } from "@stripe/stripe-js";
const stripePromise = loadStripe("your-public-key");
async function handleCheckout() {
const stripe = await stripePromise;
const { error } = await stripe.redirectToCheckout({
lineItems: [{ price: "price_id", quantity: 1 }],
mode: "payment",
successUrl: window.location.origin + "/success",
cancelUrl: window.location.origin + "/cancel",
});
if (error) {
console.error(error);
}
}
2. Handling Subscription Models
With React, managing subscriptions involves creating a subscription form and using Stripe’s API to manage recurring payments. Here’s an example of setting up a subscription flow:
javascriptCopy code// Set up a subscription with Stripe
async function createSubscription() {
const response = await fetch("/create-subscription", {
method: "POST",
headers: {
"Content-Type": "application/json",
},
});
const session = await response.json();
const { error } = await stripe.redirectToCheckout({
sessionId: session.id,
});
if (error) {
console.error(error);
}
}
Comprehensive Overview of Stripe’s Features
Subscriptions & Recurring Payments
Setting up recurring payments requires defining Products and Plans in Stripe. Using Stripe’s API, developers can:
Create subscriptions: Attach a plan to a customer.
Manage subscriptions: Update or cancel existing subscriptions.
Example in Rails:
def create_subscription
Stripe::Subscription.create(
customer: customer_id,
items: [{ price: 'price_id' }]
)
end
Charges and One-Time Payments
Handling one-time payments with Stripe is ideal for e-commerce. Stripe’s Charges API enables payments to be processed directly from your Rails or React app.
Payouts and Withdrawal Management
Stripe automates payouts, sending funds to your bank on a schedule. Payout fees vary, and managing payout settings in your dashboard allows for optimised cash flow.
Best Practices for Using Stripe Effectively
- Optimise Security: Always use Stripe’s PCI-compliant features like Stripe Elements or Stripe Checkout.
- Minimize Fees: Stripe charges a small transaction fee; consider batching payouts or using Stripe’s advanced features like Radar for fraud prevention.
- Automate Workflows: Stripe offers web-hook support to automate refunds, failed payments, and other events.
Creating an Advanced Checkout Page
The Stripe Checkout API provides a hosted payment page for simplified setup and PCI compliance. The hosted page supports Apple Pay, Google Pay, and other payment methods, enabling faster checkout for users.
In Rails, create a Checkout Session:
def create_checkout_session
session = Stripe::Checkout::Session.create(
payment_method_types: ['card'],
line_items: [{ price: 'price_id', quantity: 1 }],
mode: 'subscription',
success_url: 'https://example.com/success',
cancel_url: 'https://example.com/cancel'
)
redirect_to session.url, allow_other_host: true
end
In React, redirect to the Stripe checkout:
await stripe.redirectToCheckout({ sessionId: session.id });
Best Practices for Using Stripe Effectively
Security: Use PCI-compliant methods like Stripe Checkout or Elements for secure transactions.
Minimize Transaction Fees: Avoid unnecessary payout frequency to save on fees.
Automate Processes: Set up web-hooks to automate refunds, subscription changes, and failed payments.
Alternatives to Stripe
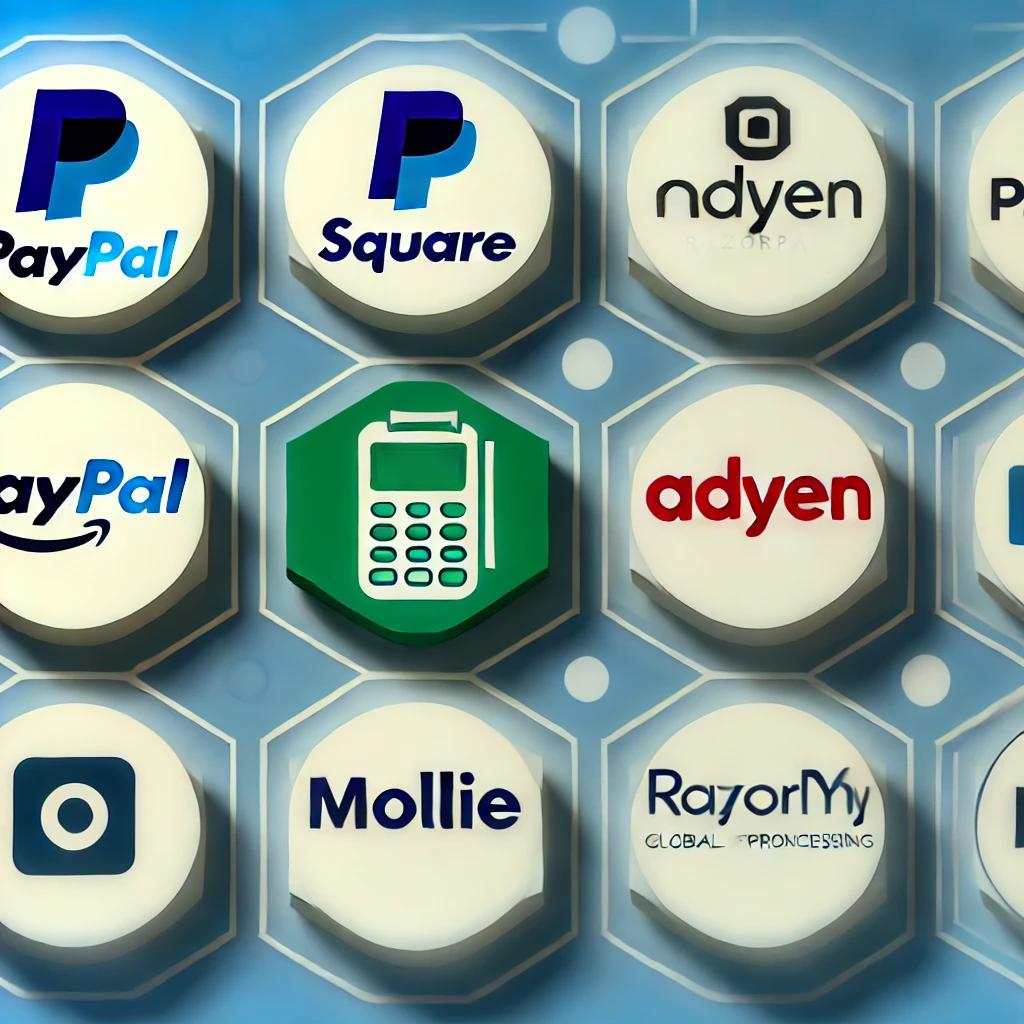
Stripe is powerful, but other payment gateways may be more suited for different regions or specific use cases:
Each of these alternatives has its strengths; for instance, PayPal is often favored for its brand recognition, while Adyen offers strong support for European merchants.
Comparison of Alternatives
Feature | Stripe | PayPal | Adyen | Mollie |
---|---|---|---|---|
Pricing | 2.9% + 30¢ | Variable | Varies | Varies |
Currencies | 135+ | 25+ | 150+ | 30+ |
Developer Tools | Excellent | Good | Good | Good |
When to Use an Alternative Payment Gateway
Alternative gateways might be better suited if your business operates in a region with limited Stripe support, or if you need specific local payment methods, such as UPI in India or Alipay in China.
Frequently Asked Questions (FAQ)
- What fees does Stripe charge? Stripe charges 2.9% + $0.30 per transaction in the US, with fees varying by country.
- How can I issue refunds? Refunds can be processed through the Stripe dashboard or programmatically via the API.
- How secure is Stripe? Stripe is PCI compliant, and it provides tools like Stripe Radar for fraud detection.
- Can I use Stripe for international payments? Yes, Stripe supports payments in 135+ currencies, making it ideal for global businesses.
Link to Stripe’s documentation and Stripe Global Availability.